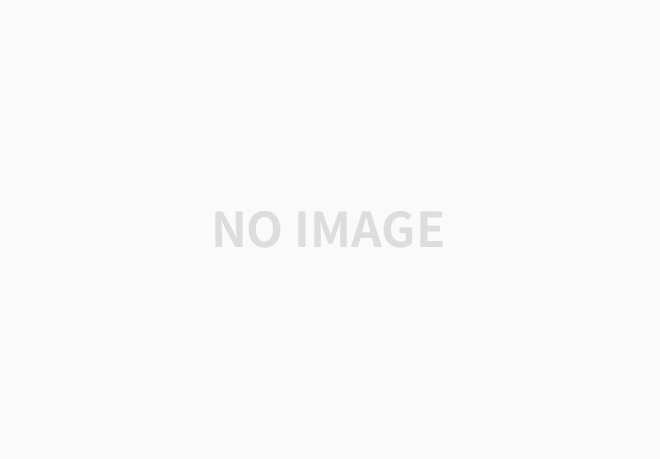
Winform에는 Z인덱스가 없더군요. 약간의 트릭을 써서 위와같은 형태를 만들 수 있었습니다.
(화면만 가려질 뿐 뒷쪽과 상호작용 가능합니다)
간단한 구조를 먼저 보자면..
1. 새로운 Form을 만들어 줍니다.
2. 새로운 Form에 WndProc 를 수정하여 통해 뒷쪽 컨트롤에게 이벤트를 넘겨줄 수 있도록 수정합니다.
3. OnPaint를 통해 문자를 그려줍니다.
4. Form의 소유자를 지정하여 소유자 상단에 위치하도록 해줍니다.
아래는 2개의 폼에 대한 코드입니다.
[Form1.cs]
using System;
using System.Drawing;
using System.Windows.Forms;
namespace DrawString
{
public partial class Form1 : Form
{
private Form2 form2 = new Form2();
public Form1()
{
InitializeComponent();
}
private void Form1_Load(object sender, EventArgs e)
{
form2.Show();
form2.Owner = this; //[4번] form1가 form2를 소유하여 상단에 위치시킵니다. (매우 중요)
pictureBox1.Image = Image.FromFile(@"C:\Users\dlaeh\Desktop\test.tif");
pictureBox1.SizeMode = PictureBoxSizeMode.Zoom;
ShowTransparentForm();
}
private void ShowTransparentForm ()
{
int offsetY = 30;
//크기에 맞춰 수정 가능합니다!
form2.Size = new Size(this.Width - 16, pictureBox1.Size.Height);
form2.Location = new Point(this.Location.X + 8,
this.Location.Y + pictureBox1.Location.Y + offsetY);
}
private void Form1_Resize(object sender, EventArgs e) //크기 변경 시 맞춰서 변경
{
ShowTransparentForm();
form2.Refresh(); //Form을 수동으로 늘리면 텍스트가 짤리더군요!
}
private void Form1_Move(object sender, EventArgs e) //이동 시에 위치 변경
{
ShowTransparentForm();
}
}
}
[Form2.cs]
using System;
using System.Drawing;
using System.Windows.Forms;
namespace DrawString
{
public partial class Form2 : Form
{
private const int WM_NCHITTEST = 0x84; //마우스 커서 위치 메시지
private const int HTTRANSPARENT = -1; //뒷쪽의 컨트롤에 메시지를 넘깁니다.
public Form2()
{
InitializeComponent();
this.Enabled = false; //상호작용 안하기 위해
this.FormBorderStyle = FormBorderStyle.None; //기본폼으로
this.Opacity = 0.5; //투명도
this.ShowInTaskbar = false; //Alt + Tab 등 선택 방지
//for test
this.BackColor = Color.Black;
}
//[2번]
//WM_NCHITTEST 와 HTTRANSPARENT 관련 공식사이트
//https://learn.microsoft.com/ko-kr/windows/win32/inputdev/wm-nchittest
protected override void WndProc(ref Message message)
{
if (message.Msg == WM_NCHITTEST) //마우스 커서 위치 메시지
{
message.Result = (IntPtr)HTTRANSPARENT; // 뒷쪽의 컨트롤에 메시지를 넘깁니다.
}
else
{
base.WndProc(ref message);
}
}
private int offsetX = 200;
private int offsetY = 300;
private int rotateOffsetY = 30;
private int rotate = 30;
protected override void OnPaint(PaintEventArgs e) //[3번] 그려주기!
{
Graphics graphics = e.Graphics;
SolidBrush myBrush = new SolidBrush(Color.FromArgb(50, 255, 50, 50));
for (int i = 0; i * offsetY + rotateOffsetY <= this.Size.Height; ++i)
{
graphics.TranslateTransform(0, i * offsetY + rotateOffsetY);
for (int j = 0; j * offsetX <= this.Size.Width; ++j)
{
graphics.TranslateTransform(offsetX, 0);
graphics.RotateTransform(-rotate);
graphics.DrawString("2024-02-11 !@#@##@ test~~", new Font(FontFamily.GenericSansSerif, 15, FontStyle.Bold), myBrush, Point.Empty);
graphics.RotateTransform(rotate);
}
graphics.ResetTransform();
}
graphics.ResetTransform();
base.OnPaint(e);
}
}
}
'C# > Windows Form' 카테고리의 다른 글
[C# Windows Form] 파일을 베이스64로 읽기 / 쓰기 (FileToBase64, Base64ToFile) (0) | 2024.02.12 |
---|---|
[C# Windows Form] Form을 Alt+Tab 에 안보이도록 설정 (0) | 2024.02.11 |
[C# Windows Form] 파일명 필터 걸기 (OpenFileDialog Filter) (0) | 2024.01.30 |
[C# Windows Form] Properties.Resources 리소스 사용하기 (1) | 2024.01.30 |
[C# Windows Form] FormBorderStyle : None 일 때 폼 크기 변경 및 움직이기 (WndProc 사용) (0) | 2024.01.27 |