부모 클래스 또는 인터페이스를 이용하여 하위 요소를 생성 및 사용할 때 이용되는 패턴입니다.
using System;
namespace ConsoleApp
{
class Program
{
public static void Main(string[] args)
{
int size = 3;
int player = 0;
Unit[] unit = new Unit[size];
while (player < size)
{
Console.WriteLine("{0}p 종족을 입력하세요: (Zerg, Protoss, Terran)", player + 1);
string race = Console.ReadLine();
switch (race)
{
case "Zerg":
unit[player] = new Zerg();
player++;
break;
case "Protoss":
unit[player] = new Protoss();
player++;
break;
case "Terran":
unit[player] = new Terran();
player++;
break;
default:
break;
}
}
Console.WriteLine();
foreach (Unit u in unit)
{
u.ShowType();
}
}
}
abstract class Unit
{
public abstract void ShowType();
}
class Zerg : Unit
{
public override void ShowType()
{
Console.WriteLine("Zerg");
}
}
class Protoss : Unit
{
public override void ShowType()
{
Console.WriteLine("Protoss");
}
}
class Terran : Unit
{
public override void ShowType()
{
Console.WriteLine("Terran");
}
}
}
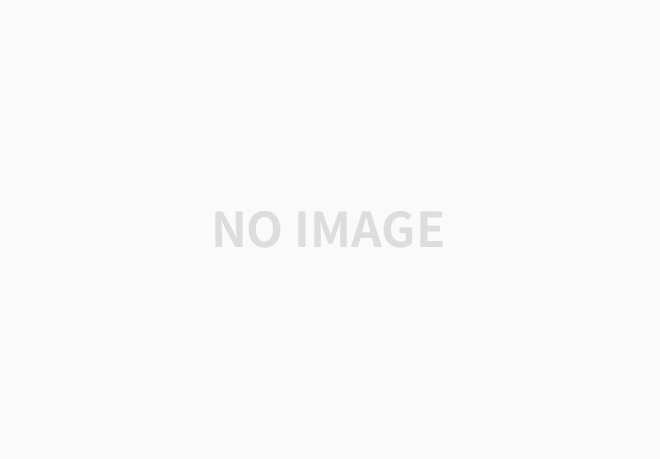
'C# > 디자인 패턴' 카테고리의 다른 글
[C# 디자인 패턴] 싱글톤 패턴 Singleton Pattern (0) | 2022.03.15 |
---|---|
[C# 디자인패턴] 옵저버 패턴 Observer Pattern (0) | 2022.03.15 |
[C# 디자인 패턴] 스트래티지 패턴 Strategy Pattern (0) | 2022.03.15 |